- Coding Vulnerability
- Secure Coding Practices
- Coding types
Common Coding Vulnerability: Essential Checks for Every Developer
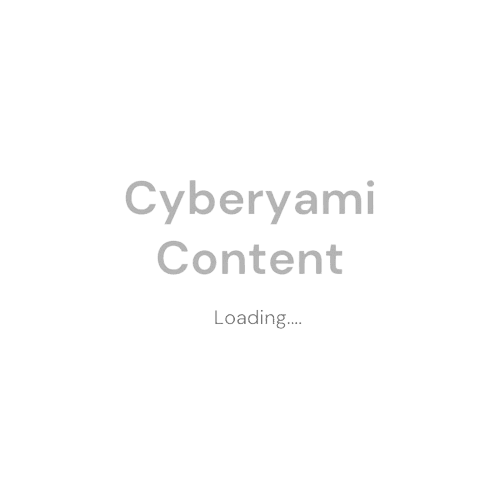
Whether you’re a programmer or a beginner you must be aware of Common Vulnerabilities in Coding & here we will check the essential vulnerabilities check that every developer should be aware of. In the vast digital landscape, the lines between innovation and exploitation blur. While programmers weave intricate threads of code, malicious actors lurk in the shadows, waiting to exploit any vulnerability. These vulnerabilities, like cracks in a digital fortress, can expose sensitive data, disrupt operations, and erode trust. Understanding these flaws and their solutions is no longer just a technical imperative, but a career-defining skill for coders navigating the ever-evolving security landscape.
What is Code Vulnerability?
A code vulnerability, also known as a software vulnerability or security vulnerability, refers to a weakness or flaw in a software system that could be exploited by attackers to compromise the system's security. These vulnerabilities can exist in various types of software, including operating systems, applications, libraries, and web applications. Let’s delve deep into Common Vulnerabilities in Coding.
Common types of code vulnerabilities include:
- Buffer Overflow:
Buffer overflow occurs when a program writes more data to a buffer (a fixed-size storage area) than it was allocated to hold. This excess data can overwrite adjacent memory, leading to unexpected behavior and potentially allowing an attacker to inject and execute malicious code.
Example: If a program expects a user to input a string of 10 characters and doesn't properly validate or handle longer inputs, an attacker could input a string longer than 10 characters, causing a buffer overflow.
2. Injection Attacks:
Injection attacks involve inserting malicious code or data into a system, usually through input fields. Common types include SQL injection, where attackers manipulate database queries, and code injection, where malicious code is injected into an application.
Example: In a web application, if user input isn't properly sanitized before being included in a database query, an attacker might input SQL commands to manipulate the database.
3. Cross-Site Scripting (XSS):
XSS occurs when attackers inject malicious scripts into web pages that are later viewed by other users. This can lead to the theft of sensitive information, session hijacking, or defacement of websites.
Example: An attacker injects a script into a comment on a blog. When other users view the comment section, the script runs in their browsers, stealing their session cookies.
4. Cross-Site Request Forgery (CSRF):
CSRF exploits the trust a web application has in a user's browser. Attackers trick users into unintentionally performing actions on a site where the user is authenticated, potentially leading to unauthorized actions.
Example: An attacker embeds a malicious link in an email or website. If a logged-in user clicks the link, it might trigger an action (e.g., changing the user's email) on a site where the user is authenticated.
5. Security Misconfigurations:
Security misconfigurations occur when software or systems are not securely configured. This can include default passwords, unnecessary services running, or overly permissive access controls.
Example: Leaving default admin passwords unchanged or exposing unnecessary services to the internet can provide easy entry points for attackers.
6. Authentication and Authorization Issues:
Weak authentication or improper authorization controls can allow unauthorized access to systems or sensitive data.
Example: Using weak passwords, not implementing multi-factor authentication, or failing to check user permissions properly can lead to unauthorized access.
7. Cryptographic Vulnerabilities:
Flaws in the implementation of cryptographic algorithms or the misuse of cryptographic functions can compromise the confidentiality and integrity of data.
Example: Using weak encryption algorithms or improperly handling cryptographic keys can expose sensitive information to attackers.
8. Denial of Service (DoS) and Distributed Denial of Service (DDoS):
DoS and DDoS attacks aim to overwhelm a system, making it unavailable to legitimate users by flooding it with excessive traffic.
Example: Sending a massive volume of requests to a website or network, exceeding its capacity, can cause it to become slow or completely unresponsive.
Addressing code vulnerabilities requires a combination of secure coding practices, regular security assessments, and prompt application of security patches and updates. This proactive approach helps minimize the risk of exploitation by malicious actors.
Top Threats: Unmasking the Common Culprits
Let's delve into the top five vulnerabilities in coding that plague the digital world:
1. Injection Flaws: Imagine malicious code injected into your input field, like a poisoned dart into a cocktail. SQL injection and Cross-site Scripting (XSS) exploit these weaknesses, allowing attackers to manipulate databases, steal data, and hijack user sessions.
Vulnerability | Description | Impact | Example |
SQL Injection | Injecting malicious code into SQL queries to manipulate databases | Unauthorized data access, modification, or deletion | Stealing customer information from an online store |
XSS | Injecting malicious scripts into web pages to exploit user browsers | Phishing attacks, stealing cookies, redirecting users | Malicious pop-up ads appearing on a legitimate website |
2. Broken Authentication: The gatekeeper asleep on the job! Weak authentication mechanisms, like default passwords or insecure password hashing, grant unauthorized access, allowing attackers to impersonate legitimate users and wreak havoc.
Vulnerability | Description | Impact | Example |
Weak Passwords | Using predictable or easily guessable passwords | Account takeover, unauthorized access to sensitive data | Hacking into user accounts with common passwords like "123456" |
Insecure Hashing | Storing passwords without secure hashing algorithms | Password exposure, credential theft | Leaked password databases enabling attackers to crack user passwords |
3. Access Control Lapses: Picture a bank vault with open doors. Inadequate access control mechanisms grant inappropriate access to unauthorized users, exposing sensitive data and compromising system integrity.
Vulnerability | Description | Impact | Example |
Missing Authorization | Lack of checks to ensure users have permission to access specific data or resources | Unauthorized data access, modification, or deletion | An employee in a financial institution accessing customer accounts they shouldn't |
Improper Role Management | Assigning incorrect roles or privileges to users | Granting excessive permissions, enabling misuse of resources | A customer service representative having admin privileges in a database |
4. Cryptographic Failures: When the encryption key falls into the wrong hands, the message becomes clear - your data is exposed. Weak encryption algorithms, insecure key management, and hardcoded secrets can all lead to data breaches.
Vulnerability | Description | Impact | Example |
Weak Encryption Algorithms | Using outdated or insufficiently strong encryption algorithms | Data decryption, information exposure | Sensitive data like credit card numbers being intercepted in transit |
Insecure Key Management | Poorly storing or managing encryption keys | Unauthorized access to keys, decryption of encrypted data | Leaked encryption keys allowing attackers to decrypt confidential information |
5. Insecure Direct Object References (IDOR): Imagine a treasure map leading directly to hidden riches. IDOR vulnerabilities expose sensitive data by revealing direct references to objects, allowing attackers to bypass access controls and access unauthorized information.
Vulnerability | Description | Impact | Example |
Predictable Object IDs | Using predictable or guessable identifiers for objects | Unauthorized access to sensitive data based on IDs | Attackers accessing customer records by guessing sequential IDs |
Missing Access Control Checks | Failing to check user permissions before exposing object details | Bypassing access controls, unauthorized data access | An attacker accessing another user's profile information |
How to avoid Coding Vulnerabilities ?
Avoiding coding vulnerabilities involves adopting secure coding practices throughout the software development lifecycle. Here are detailed steps and principles to help mitigate the risk of vulnerabilities:
- Secure Coding Standards:
Establish and adhere to secure coding standards for the programming languages and frameworks you are using. These standards should cover input validation, data sanitization, proper error handling, and secure use of libraries and APIs.
Implementation: Create a set of coding guidelines that developers must follow. Regularly update these standards based on emerging threats and best practices.
2. Input Validation and Data Sanitization:
Validate and sanitize all user inputs to prevent injection attacks, such as SQL injection or cross-site scripting (XSS).
Implementation: Use input validation functions and libraries to ensure that input conforms to expected formats and lengths. Employ parameterized queries for database interactions to prevent SQL injection.
3. Buffer Overflow Protection:
Implement measures to prevent buffer overflow vulnerabilities.
Implementation: Use safer functions and libraries that automatically handle buffer sizes. If possible, use programming languages that provide memory safety features, like bounds checking.
4. Code Reviews:
Regularly conduct thorough code reviews to identify and address security issues.
Implementation: Establish a systematic code review process where developers and peers review each other's code for security vulnerabilities. Include security experts in the review process when possible.
5. Static Analysis Tools:
Utilize static analysis tools to automatically scan code for potential vulnerabilities.
Implementation: Integrate static analysis tools into your development pipeline to identify issues early in the development process. Address the identified vulnerabilities promptly.
6. Dynamic Analysis and Penetration Testing:
Conduct dynamic analysis and penetration testing to identify vulnerabilities in a running application.
Implementation: Regularly perform penetration tests and dynamic analysis on your application. Test for common vulnerabilities, such as injection attacks, cross-site scripting, and insecure configurations.
7. Secure Authentication and Authorization:
Implement strong authentication mechanisms and proper authorization controls.
Implementation: Enforce the use of strong, unique passwords. Implement multi-factor authentication where possible. Ensure that users have the minimum necessary permissions to perform their tasks, following the principle of least privilege.
8. Security Misconfigurations:
Regularly audit and address security configurations to avoid misconfigurations.
Implementation: Develop a checklist for secure configurations and review the configuration settings of servers, databases, and other components regularly. Automated tools can help identify and correct misconfigurations.
9. Cryptographic Best Practices:
Follow cryptographic best practices to ensure the confidentiality and integrity of data.
Implementation: Use well-established cryptographic algorithms and libraries. Regularly update cryptographic libraries and algorithms to patch any vulnerabilities. Implement proper key management practices.
10. Continuous Education and Training:
Keep developers, as well as other team members, educated about the latest security threats and best practices.
Implementation: Provide regular security training for developers, covering secure coding practices, emerging threats, and common vulnerabilities. Encourage ongoing learning and awareness.
11. Patch Management:
Keep all software components up to date with the latest security patches.
Implementation: Regularly check for security updates and patches for the programming languages, frameworks, libraries, and other software components used in your application. Apply patches promptly to address known vulnerabilities.
12. Incident Response Plan:
Have an incident response plan in place to address and mitigate security incidents promptly.
Implementation: Develop a clear incident response plan outlining the steps to take in the event of a security breach. Regularly review and update the plan and conduct drills to ensure a swift and effective response.
By integrating these practices into the software development lifecycle, organizations can significantly reduce the risk of coding vulnerabilities and enhance the overall security posture of their applications. Security should be an integral part of the development process, from design to deployment and ongoing maintenance.
What types of Coding Challenges are there?
Coding challenges are problems or puzzles that require a person to write a program to solve them. They are commonly used in technical interviews, coding competitions, and online platforms to assess a programmer's problem-solving skills, algorithmic knowledge, and coding proficiency. These challenges come in various difficulty levels and cover a wide range of topics, including data structures, algorithms, mathematics, and more.
Here are some common coding Types challenges and a brief explanation of each:
Algorithmic Challenges:
- Sorting Algorithms: Implementing sorting algorithms like bubble sort, quicksort, or merge sort.
- Searching Algorithms: Implementing search algorithms like binary search or linear search.
- Dynamic Programming: Solving problems using dynamic programming techniques to optimize solutions.
Data Structure Challenges:
- Array Manipulation: Solving problems that involve manipulating arrays, such as finding subarrays with specific properties.
- Linked List Problems: Implementing or solving problems related to linked lists.
- Tree and Graph Problems: Solving problems involving binary trees, graphs, and related algorithms.
Mathematical Challenges:
- Number Theory: Solving problems related to prime numbers, factorization, or other number theory concepts.
- Combinatorics: Problems involving combinations, permutations, and counting principles.
- Geometry: Solving geometric problems that require mathematical reasoning.
String Manipulation Challenges:
- String Algorithms: Implementing algorithms for string matching, parsing, or manipulation.
- Regular Expressions: Solving problems that involve the use of regular expressions.
Dynamic Programming Challenges:
- Longest Common Subsequence (LCS): Finding the longest subsequence common to two sequences.
- Knapsack Problem: Solving optimization problems where items have associated weights and values.
Graph Problems:
- Shortest Path Algorithms: Implementing algorithms like Dijkstra's or Floyd-Warshall for finding the shortest path in a graph.
- Graph Traversal: Solving problems related to depth-first search (DFS) or breadth-first search (BFS).
System Design Challenges:
- Design Patterns: Implementing or solving problems using common design patterns.
- Object-Oriented Design: Designing systems with a focus on object-oriented principles.
Concurrency Challenges:
- Multithreading Problems: Solving problems that involve concurrent programming and synchronization.
Database Challenges:
- SQL Problems: Writing SQL queries to solve specific problems or retrieve data from databases.
Online Coding Platforms:
- Websites like LeetCode, HackerRank, and CodeSignal provide a variety of challenges across different categories and difficulty levels.
So these are some of Coding types that you face. When facing coding challenges, it's important to analyze the problem, devise an efficient algorithm, and implement a solution using a programming language of your choice. The goal is not only to produce a correct solution but also to write clean, readable, and efficient code.
The Importance of Secure Coding Practices
Protection Against Cyber Threats
Secure coding practices are essential for protecting software applications from a wide range of cyber threats. By addressing vulnerabilities early in the development process, developers can create robust and resilient systems that are less susceptible to attacks.
Maintaining User Trust
In an era where data breaches are increasingly common, users are more concerned about the security of their information. Secure coding instills confidence in users, fostering trust and loyalty. Applications with a strong security foundation are more likely to be adopted and recommended.
Regulatory Compliance
Many industries are subject to stringent data protection regulations. Adhering to secure coding practices is not just good practice; it is often a legal requirement. Compliance with regulations such as GDPR, HIPAA, or PCI DSS is crucial for avoiding penalties and legal consequences.
The Future of Secure Coding
Integration of AI and Machine Learning in Code Analysis
As technology advances, the integration of AI and machine learning in code analysis is becoming more prevalent. Automated tools can identify patterns, detect anomalies, and predict potential vulnerabilities, streamlining the code review process and enhancing overall security.
DevSecOps Practices
DevSecOps, the integration of security into the DevOps process, is gaining traction. By incorporating security measures throughout the development lifecycle, DevSecOps aims to create a culture where security is not a separate phase but an integral part of the development process.
Emphasis on Education and Training
As the importance of secure coding becomes more evident, there is a growing emphasis on educating developers about security best practices. Training programs and certifications are available to help developers acquire the necessary skills to write secure code and identify potential vulnerabilities.
Career Opportunities in Secure Coding
Job Roles
Security Engineer:
- Responsibilities include identifying and addressing security vulnerabilities in software applications, conducting security assessments, and implementing security measures.
Penetration Tester:
- Conducts ethical hacking to identify vulnerabilities in systems and applications, simulating real-world cyber-attacks to assess security posture.
Secure Code Reviewer:
- Specializes in reviewing code to identify and rectify security flaws, ensuring that applications adhere to secure coding practices.
Skill Sets and Qualifications
Programming Languages:
- Proficiency in languages such as Java, C++, Python, and JavaScript is crucial for writing secure code.
Security Knowledge:
- Understanding of security principles, encryption, authentication, and authorization mechanisms is essential.
Certifications:
- Certifications like CEH, CSSLP, and CISSP demonstrate expertise in ethical hacking, secure software development, and information security.
🔒 The Demand for Secure Coding Experts is Skyrocketing! 🔒
The demand for secure coding experts is rapidly increasing as businesses prioritize cybersecurity. Coding is an essential part of cybersecurity, promising great career opportunities for those seeking to enter the cybersecurity domain.
🌟 Introducing the Cyberyami Certified Secure Coding Expert (C|CSCE) certification – your gateway to becoming a recognized leader in secure coding practices!
🚀 Why Choose C|CSCE?
1. Advanced Skill Set
2. Industry Recognition
3. Enhanced Career Opportunities
4. Compliance Assurance
5. Future-Proof Your Skills
🔑 Join the Elite League of Secure Coding Experts!
Don't miss out on the opportunity to boost your career and make a significant impact in cybersecurity. Enroll in the C|CSCE certification program today and take your coding skills to the next level!
Conclusion
In conclusion, understanding and addressing common vulnerabilities in coding is paramount for building secure and resilient software applications. The solutions discussed in this blog, coupled with the importance of secure coding practices offer a robust framework for developers to create systems that can withstand cyber threats. The future of secure coding looks promising, with advancements in AI, the integration of security into DevOps practices, and a growing emphasis on education and training. Now you must have been cleared with Coding Types, Challenges & importance of Secure of Coding. For those considering a career in secure coding, the diverse job roles, certification programs, and required skill sets provide a pathway to a rewarding and impactful profession in the ever-evolving field of cybersecurity.