- learn python
- python programming interview
- how to became python programmer
Cracking the Python Programming Interview: Common Questions & Solutions
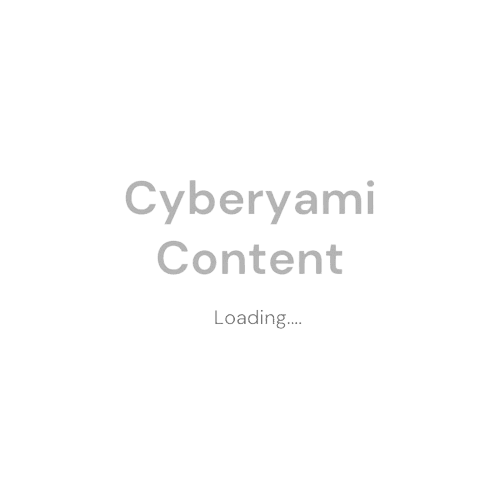
Python has gained immense popularity as a versatile programming language used in various domains, from web development to data analysis. As a Python developer, it's essential to be well-prepared for technical interviews that assess your skills and knowledge. In this blog, we will explore some common Python interview questions and provide solutions to help you succeed in your next interview.
What is Python and Why is it Popular?
Python is a high-level, interpreted programming language known for its simplicity and readability. It offers a wide range of libraries and frameworks, making it suitable for diverse applications. Its popularity stems from its ease of use, extensive community support, and broad adoption across industries.
One of the key reasons for Python's popularity is its ease of use. Its syntax is designed to be intuitive and expressive, allowing developers to write clean and concise code. This makes Python a great language for beginners who are just starting their programming journey. With its readable and understandable syntax, newcomers can quickly grasp the fundamentals of programming and begin building their own projects.
Python is also a highly versatile language, with a vast ecosystem of libraries and frameworks that extend its capabilities. The Python Package Index (PyPI) houses thousands of open-source libraries that cover a wide range of domains, including web development, data analysis, machine learning, and more. This extensive collection of libraries empowers developers to leverage existing solutions and accelerate their development process.
Key Python Fundamentals
To excel in a Python interview, it's crucial to have a strong grasp of the fundamentals. Familiarize yourself with concepts like data types, variables, control structures, loops, functions, and object-oriented programming (OOP) principles. Understanding these fundamentals will lay a solid foundation for tackling more complex questions.
- Syntax and Data Types: Python has a clean and readable syntax that makes it easy to write and understand code. It uses indentation to define blocks of code, which enhances code readability. Python supports various data types, including integers, floats, strings, lists, tuples, dictionaries, and more. Understanding how to work with these data types is essential for manipulating and storing data in your programs.
- Variables and Operators: In Python, variables are used to store data values. They are created with a specific name and assigned a value. Python supports a wide range of operators, including arithmetic, assignment, comparison, logical, and more. Understanding how to declare variables and utilize operators is essential for performing calculations and making logical decisions in your programs.
- Control Flow: Control flow refers to the order in which statements are executed in a program. Python provides control flow structures such as if-else statements, loops (for and while), and conditional expressions (ternary operators). These structures allow you to control the flow of your program based on certain conditions or iterate over a sequence of values.
- Functions: Functions are reusable blocks of code that perform a specific task. They allow you to encapsulate functionality, promote code reusability, and improve code organization. In Python, you can define functions using the "def" keyword, specify parameters, and return values. Understanding how to define and use functions is crucial for writing modular and efficient code.
- File Handling: Python provides built-in functions and modules for reading from and writing to files. File handling is essential for working with data stored in external files, such as text files, CSV files, JSON files, and more. Understanding how to open, read, write, and close files is important for managing data persistence and interacting with the file system.
- Exception Handling: Exception handling allows you to handle and manage errors that may occur during program execution. Python provides a try-except block for catching and handling exceptions. Understanding how to handle exceptions gracefully helps you write robust and reliable code.
- Object-Oriented Programming (OOP): Python supports object-oriented programming, which enables you to create and define classes, objects, and methods. OOP allows for the organization of code into reusable and modular structures, enhancing code maintainability and reusability.
Python Interview Questions for Beginners
For entry-level positions, interviewers often assess your understanding of basic Python concepts. Some common questions for beginners include:
- What is Python, and why is it popular?
- Python is a high-level programming language known for its simplicity and readability. It has a large and active community, extensive library support, and is widely used in various domains such as web development, data analysis, artificial intelligence, and more.
- What are the key features of Python?
- Some key features of Python include its easy-to-read syntax, dynamic typing, automatic memory management, extensive standard library, and support for multiple programming paradigms (procedural, object-oriented, functional).
- What are the differences between Python 2 and Python 3?
- Python 2 and Python 3 are two major versions of Python. Python 3 introduced several changes and improvements over Python 2, including better Unicode support, print function as a built-in function, improved syntax, and more. Python 2 is no longer actively developed or supported.
- Explain the concept of Python's Global Interpreter Lock (GIL).
- The Global Interpreter Lock (GIL) is a mechanism in CPython (the reference implementation of Python) that allows only one thread to execute Python bytecodes at a time. This limitation can impact the performance of multithreaded Python programs, particularly in CPU-bound tasks. However, it doesn't affect the execution of I/O-bound tasks or programs utilizing multiple processes.
- What are the main differences between a list and a tuple in Python?
- A list is a mutable sequence that allows adding, removing, and modifying elements. It is denoted by square brackets ([]). In contrast, a tuple is an immutable sequence that cannot be modified once created. It is denoted by parentheses (()).
- What is the purpose of the "if name == 'main':" statement?
- The "if name == 'main':" statement is used to check if the current script is being run directly (as the main module) or being imported as a module in another script. It allows you to include code that should only run when the script is executed directly.
- How do you handle exceptions in Python?
- Exceptions in Python can be handled using a try-except block. The code inside the try block is executed, and if an exception occurs, it is caught by the corresponding except block. Exception handling allows for graceful error handling and prevents program termination.
Python Interview Questions for Experienced Developers
As you progress in your career, the interview questions become more challenging. Here are some topics that experienced Python developers should be prepared for:
- What are decorators in Python?
- Decorators in Python are a way to modify the behavior of a function or class without directly modifying its source code. They allow you to wrap a function or class with additional functionality, such as logging, timing, or authentication, by using the @decorator_name syntax.
- Explain the concept of generators in Python.
- Generators are a type of iterable in Python that generate values on-the-fly instead of storing them in memory. They are defined using the yield keyword and allow you to iterate over a sequence of values without the need to create the entire sequence beforehand. This can be useful when working with large datasets or when memory efficiency is a concern.
- What is the difference between shallow copy and deep copy in Python?
- Shallow copy and deep copy are methods to create copies of objects in Python. Shallow copy creates a new object that references the same memory locations as the original object, while deep copy creates a completely independent copy with its own memory locations. Modifying a deeply copied object does not affect the original object, whereas modifying a shallow copied object may impact the original object.
- What are the differences between a module and a package in Python?
- In Python, a module is a single file that contains Python code, while a package is a collection of modules organized in a directory hierarchy. Packages are used to create a modular and organized structure for Python projects. Modules can be imported and used within other modules or scripts.
- Explain the concept of name mangling in Python.
- Name mangling is a feature in Python that adds a prefix of double underscores (__) to attribute names to make them unique within a class. This helps to avoid naming conflicts when subclassing and accessing attributes from outside the class. Name mangling is primarily used to indicate that an attribute is intended to be private to the class.
- What are metaclasses in Python?
- Metaclasses are classes that define the behavior of other classes. They allow you to customize the creation and behavior of classes at runtime. Metaclasses are used less frequently compared to regular classes but can be powerful tools for advanced use cases, such as framework development or implementing custom class behavior.
- How does garbage collection work in Python?
- Python uses automatic garbage collection to reclaim memory occupied by objects that are no longer referenced or used in the program. The garbage collector periodically identifies and frees up memory occupied by unused objects. The specific algorithm used for garbage collection in Python is called reference counting, supplemented by a cycle detection mechanism called garbage collection.
Python Coding Challenges and Problem-Solving
Coding challenges are often a part of technical interviews. Interviewers may present you with a problem and assess your ability to write efficient and clean Python code to solve it. Practice solving algorithmic problems and familiarize yourself with common data structures like lists, dictionaries, and sets.
Preparing for the Python Interview
To succeed in a Python interview, thorough preparation is key. Here are some tips to help you prepare effectively:
- Review important Python concepts and syntax.
- Solve coding problems from online platforms like LeetCode and HackerRank.
- Practice answering interview-style questions and explaining your thought process.
- Research the company and the role to tailor your preparation to their specific needs.
Conclusion
Cracking a Python programming interview requires a combination of knowledge, problem-solving skills, and effective communication. By understanding the fundamentals, practicing coding challenges, and being well-prepared, you can confidently approach Python interviews and showcase your expertise. Remember to keep learning and refining your skills to stay competitive in the ever-evolving field of Python development.
Incorporating the provided keywords: python interview questions, learn python, python for beginners, free python course, python online course, Python tools, python programming for beginners, programming languages, python fundamentals, python coding for interview, python interview questions for experience, python interview questions for freshers.